6257.删除每行中的最大值
给你一个 m x n
大小的矩阵 grid
,由若干正整数组成。
执行下述操作,直到 grid
变为空矩阵:
- 从每一行删除值最大的元素。如果存在多个这样的值,删除其中任何一个。
- 将所有被删除元素中的最大值相加。
每执行一次操作,矩阵中列的数据量就会减1
返回执行上述操作后的答案
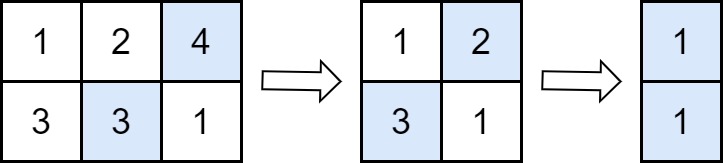
1 2 3 4 5
| 上图展示在每一步中需要移除的值。 - 在第一步操作中,从第一行删除 4 ,从第二行删除 3(注意,有两个单元格中的值为 3 ,我们可以删除任一)。在答案上加 4 。 - 在第二步操作中,从第一行删除 2 ,从第二行删除 3 。在答案上加 3 。 - 在第三步操作中,从第一行删除 1 ,从第二行删除 1 。在答案上加 1 。 最终,答案 = 4 + 3 + 1 = 8 。
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37
| class Solution { public int deleteGreatestValue(int[][] grid) { int res = 0; int n = grid[0].length; for(int i=0;i<n;i++){ int max = Integer.MIN_VALUE; for(int[] temp: grid){ Pair p = findMax(temp); max = Math.max(max,p.val); temp[p.i]=0; } res+=max; } return res; } Pair findMax(int[] arr){ PriorityQueue<Pair> pq = new PriorityQueue<>(new Comparator<Pair>(){ public int compare(Pair o1, Pair o2) { return o2.val - o1.val; } }); for(int i=0;i<arr.length;i++){ pq.add(new Pair(i,arr[i])); } return pq.peek(); } } class Pair{ int i; int val; public Pair(int i, int val){ this.i = i; this.val = val; } }
|
2487.从链表中移除节点
给你一个链表的头节点 head
。
对于列表中的每个节点 node
,如果其右侧存在一个具有 严格更大 值的节点,则移除 node
。
返回修改后链表的头节点 head
。

1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| class Solution { public ListNode removeNodes(ListNode head) { ArrayDeque<ListNode> ad = new ArrayDeque(); ListNode dummy = new ListNode(-1); ListNode temp = dummy; while(head!=null){ while(!ad.isEmpty()){ if(ad.peek().val<head.val) ad.pop(); else break; } ad.push(head); head=head.next; } while(!ad.isEmpty()){ temp.next = ad.removeLast(); temp=temp.next; } return dummy.next; } }
|